API is designed for create modules and write modifications that are compatible with older and future versions of Datalife Engine script. If you use an API to retrieve or query data from the database, you can be sure that the code will work in future versions, so you can create and alter testing module and adapt the code to use with a new version. Also, when using the API you don't need to write your own functions to retrieve data, there is no need to include and declare needed to work with the database classes. Simply include the API file with your module and start using its features, everything else will work just fine and functions of the classes will control your modules to get it to fit together.
dlestarter
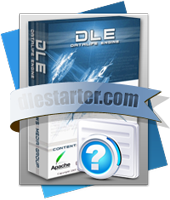
API is designed for create modules and write modifications that are compatible with older and future versions of Datalife Engine script. If you use an API to retrieve or query data from the database, you can be sure that the code will work in future versions, so you can create and alter testing module and adapt the code to use with a new version. Also, when using the API you don't need to write your own functions to retrieve data, there is no need to include and declare needed to work with the database classes. Simply include the API file with your module and start using its features, everything else will work just fine and functions of the classes will control your modules to get it to fit together.
To use the API in module, you have to include the line of code to your module file:
include ('engine/api/api.class.php');
In this case you do not need to worry whether there is a connection to the database or not connected or include required classes or not. This code you can use inside the files within DLE and just the other scripts that are not related to DLE. This what you can organize the standard integration of DLE with other scripts ex. forums.
List of API functions:
Develop a full API, and only started at the moment until API has a limited set of functions. We therefore ask all those interested in this API developers to visit this topic and leave your suggestions on what new features you want to see in new versions. Because only based on your needs, you can create a full API to cover all functions and all aspects of your request and features.